PROJECT: SecureIT
My team of 4 software engineering students and I were tasked with enhancing a basic command line interface desktop addressbook application for our Software Engineering project. We chose to morph it into a password manager, SecureIT. Our app allows businesses to securely store and manage their confidential information, allowing employees to easily retrieve such information if required.
My role was to implement the cards management feature of our app. This is how the cards mode looks like:
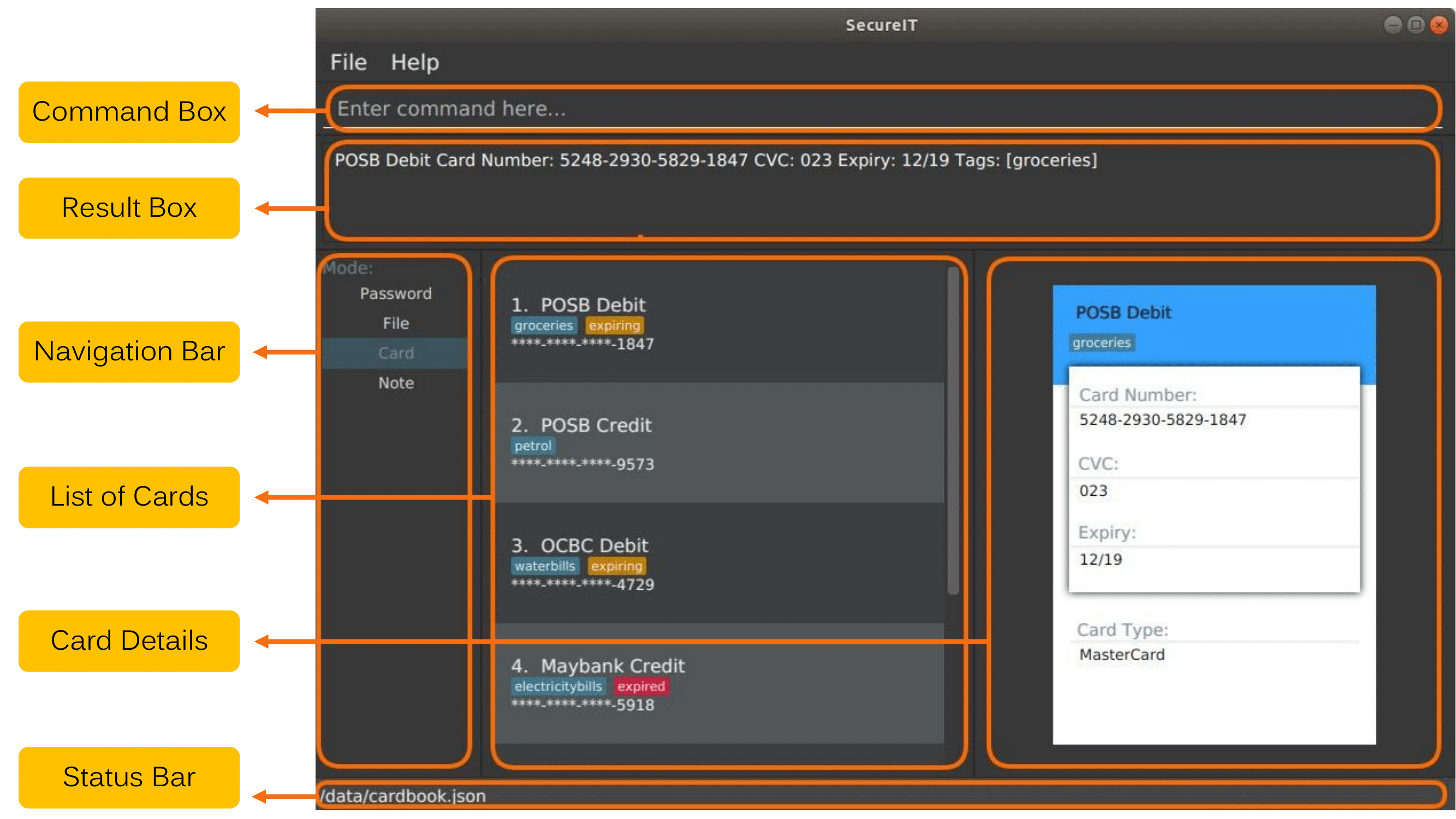
Note that the following symbols and formatting used in this documents:
Icon |
Meaning |
![]() |
Some tips for you to familiarise with the commands |
![]() |
Warning! Danger zone. Make sure you know what you are doing |
Summary of contributions
-
Major enhancement: added card features to the app
-
What it does: allows the user to store credit card details securely and retrieve them easily in the app
-
Justification: In an app that stores confidential data, credit card details are definitely data that users need to keep secure. The cards feature allows users to easily retrieve their card details and take the necessary actions when their card is about to expire.
-
Highlights: This feature also sends notifications to users when their cards are about to expire. There is also an automatic tagging system displayed in the cards list when cards have expired or are about to expire.
-
-
Minor enhancement: Refactored
CommandResult
constructors to use theBuilder
class, to allow us to customise our constructors, thus minimizing the amount of code required for various constructors. -
Code contributed: [Functional code]
-
Other contributions
-
Project management:
-
Enhancements to existing features:
-
Refactor CommandResult class (Pull request #73)
-
-
Documentation:
-
Community:
-
Contributions to the User Guide
Given below are sections that I have contributed to the updated User Guide, with additions regarding the card feature that I had implemented. They showcase my ability to write documentation targeting end-users. |
Card
Too many credit cards to carry around? With SecureIT, you can easily manage your card information.
To access your cards, simply enter goto card
in the command box.
For the following commands, simply follow the format given and type the command into the command box. Press Enter
to execute it.
Creating a card : add
You can easily adds cards to the application.
Let’s say that you have just gotten a fresh new credit card from the bank and you want to add it into your cards list.
You can type in the command.
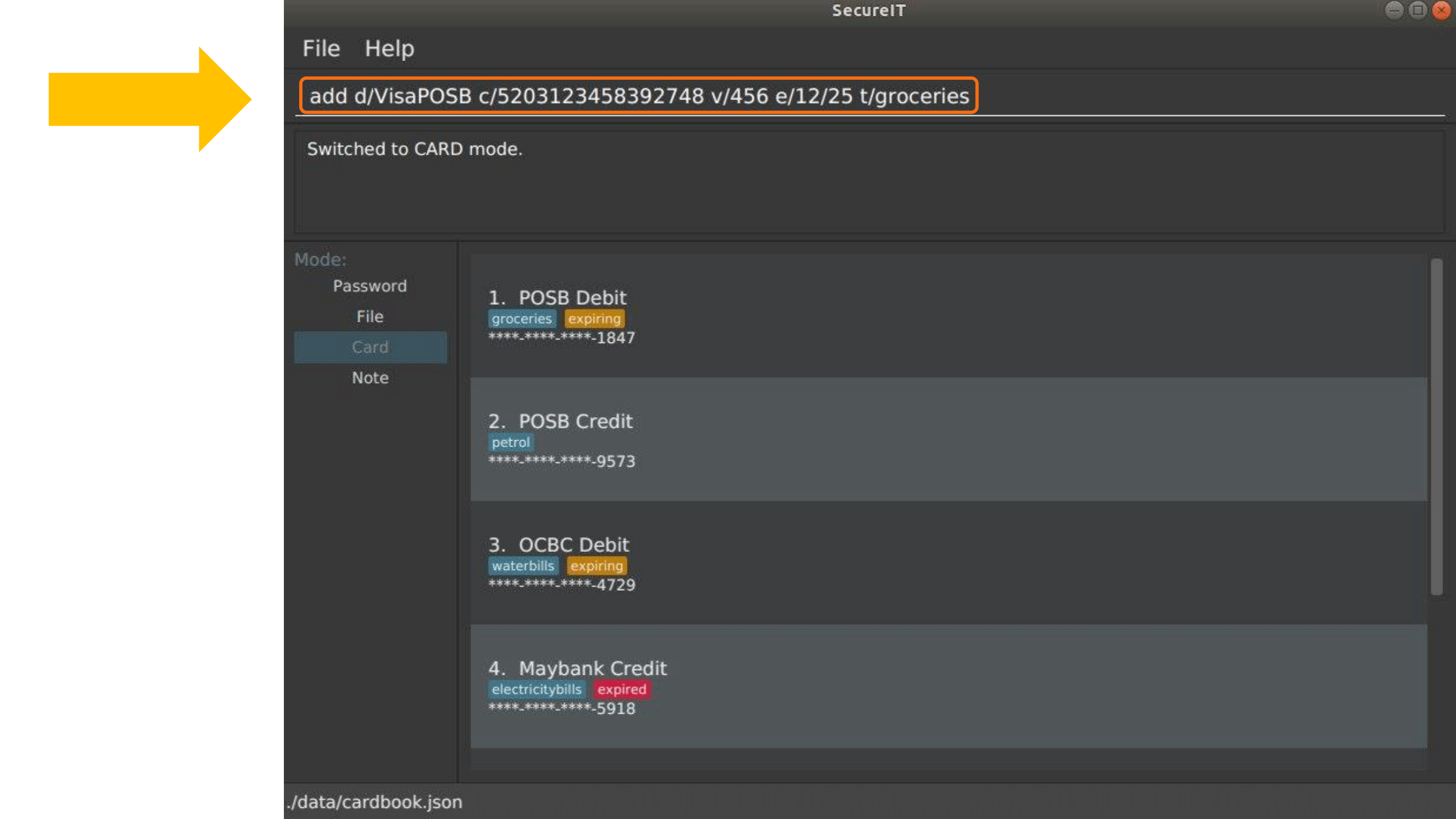
Format: add d/DESCRIPTION c/CARDNUMBER v/CVC e/EXPIRY [t/TAG]
Example: add d/VisaPOSB c/5203123458392748 v/456 e/12/25 t/groceries
The result box will display the message as shown. You can see that your card has been successfully added into the list.
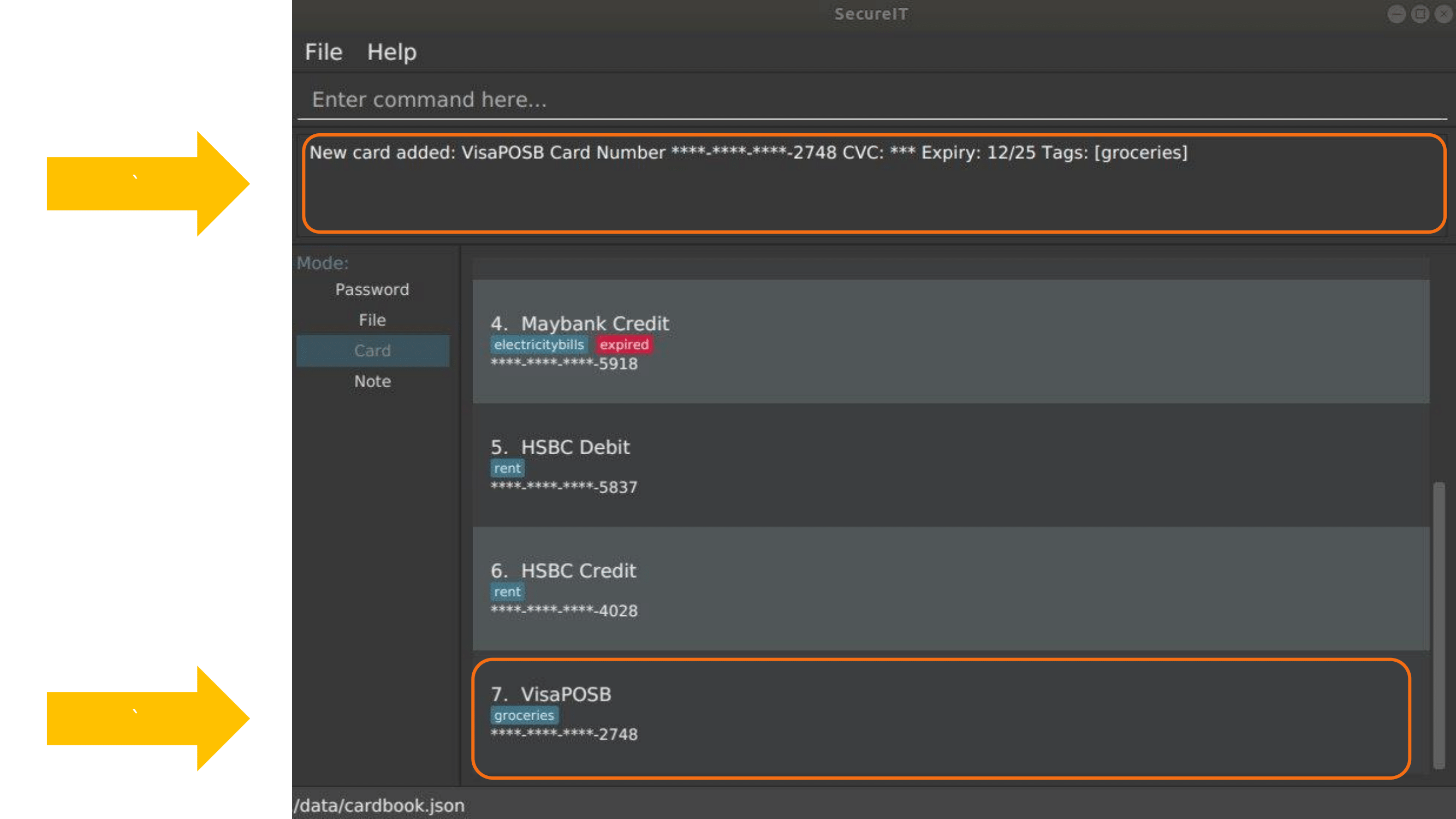
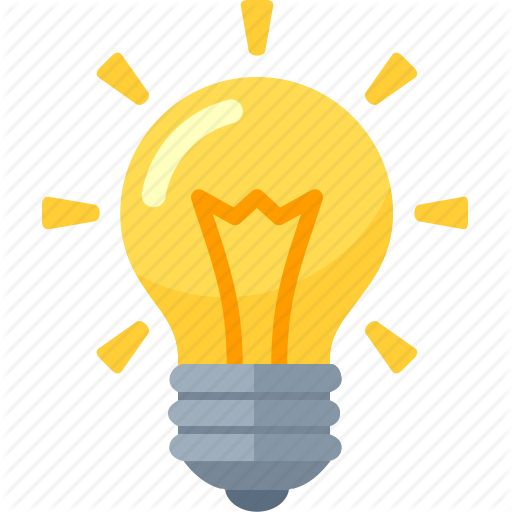
Format:
-
Description entered is case sensitive.
-
Card numbers can be entered with or without dashes.
-
With dashes: 1234-5678-1234-5678
-
Without dashes: 1234567812345678
-
-
Card expiry is of the format MM/YY.
-
You can include up to 5 tags.
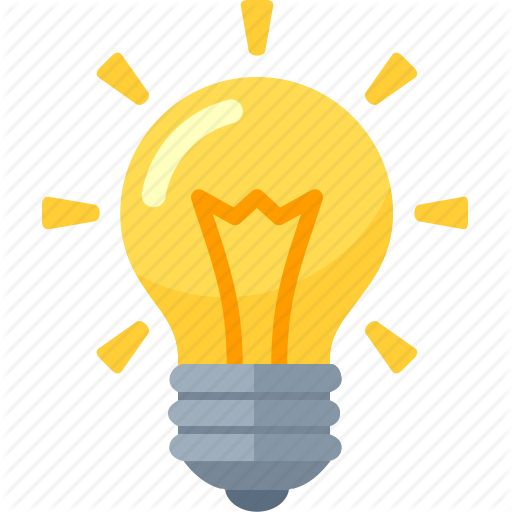
Note:
-
SecureIT does not support cards types that are not MasterCard or Visa.
-
All card numbers entered must have 16 digits.
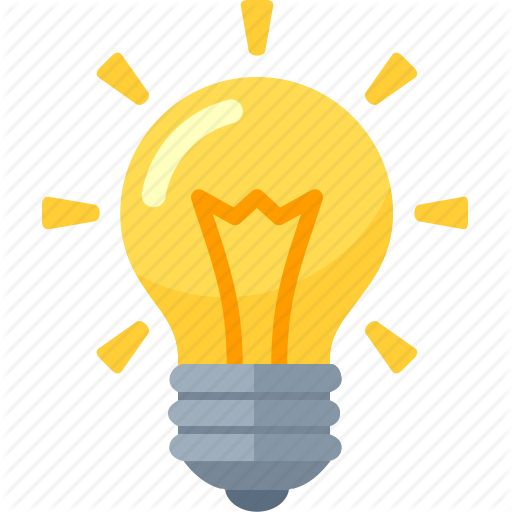
Tip:
-
After adding your cards, a card’s description and its index in the list are interchangeable with each other. In other words, the following commands accept either description or index, but not both.
-
If you know exactly which card you need, you can easily type the description without needing to scroll through the list.
-
When your card is about the expire, SecureIT warns you with a notification upon start up. On top of that, you can easily see which cards are expiring or expired in the cards list.
Reading a card : read
If you already have a card in mind and want to view its details, you can easily do so by entering the card description and its CVC. You can also obtain the same result by using a card’s index in the list with the correct CVC.
Format: read d/DESCRIPTION v/CVC
OR read INDEX v/CVC
Example: read d/VisaPOSB v/456
OR read 1 v/456
Type in the command.
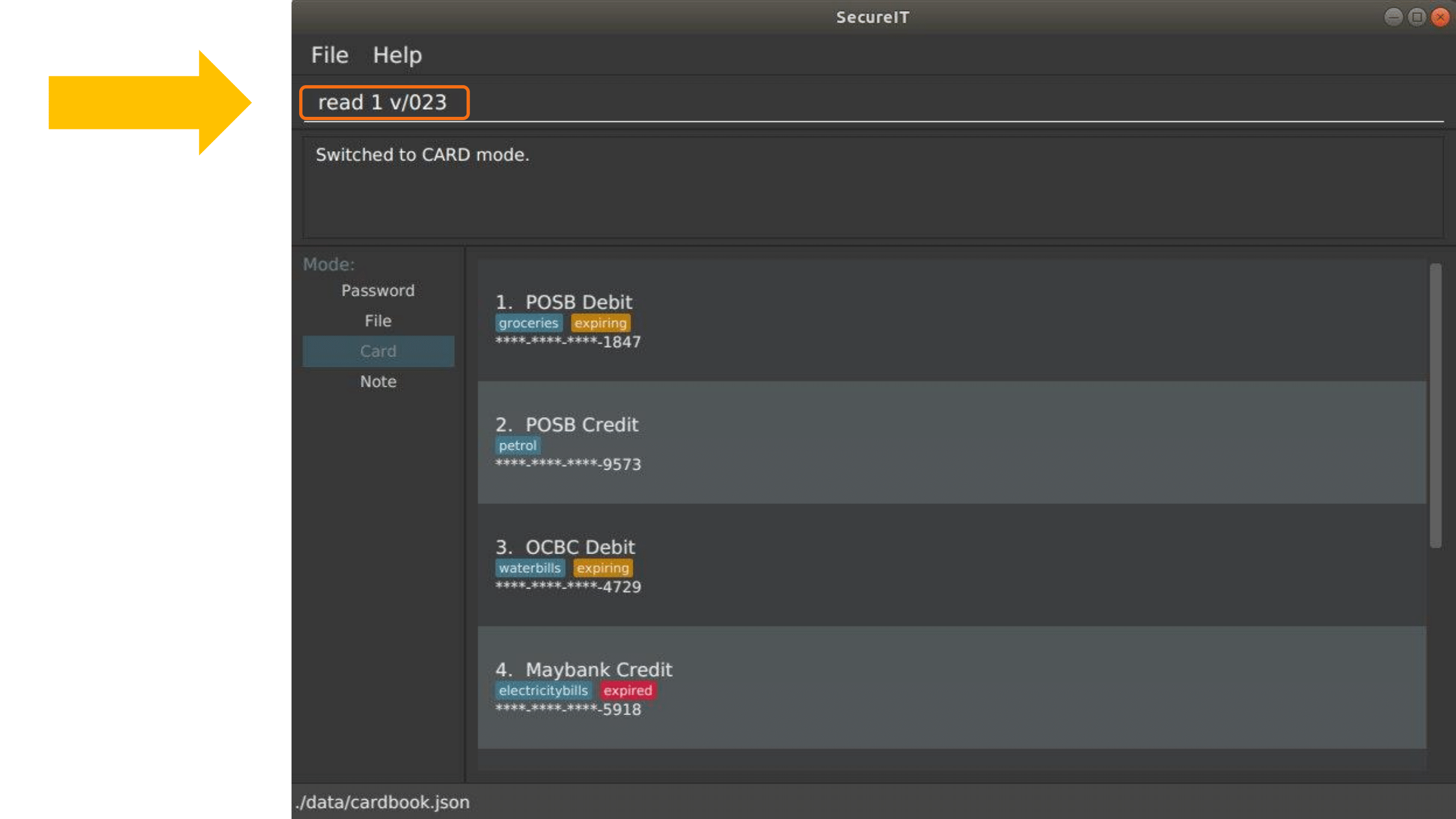
The result box will display the message as shown. You can see a new panel that contains your desired credit card details appearing at the side.
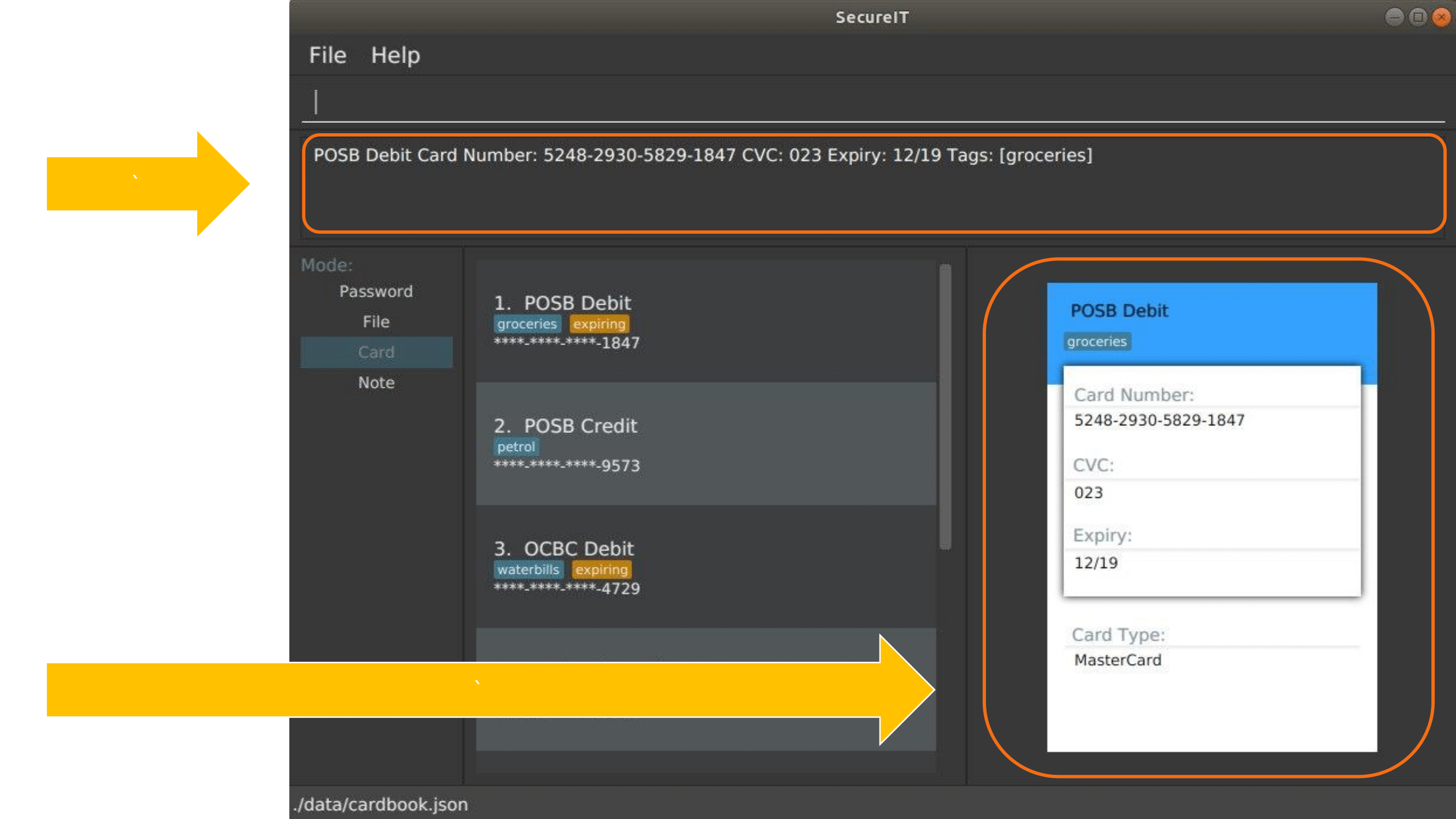
Copying a card : copy
You can copy a specific card’s card number to your clipboard as well.
You can choose to copy the card number using its description. Alternatively, you can also perform the command with the card’s index in the list:
Format: copy d/DESCRIPTION v/CVC
OR copy INDEX v/CVC
Example: copy d/VisaPOSB v/456
OR copy 1 v/456
Type in the command.
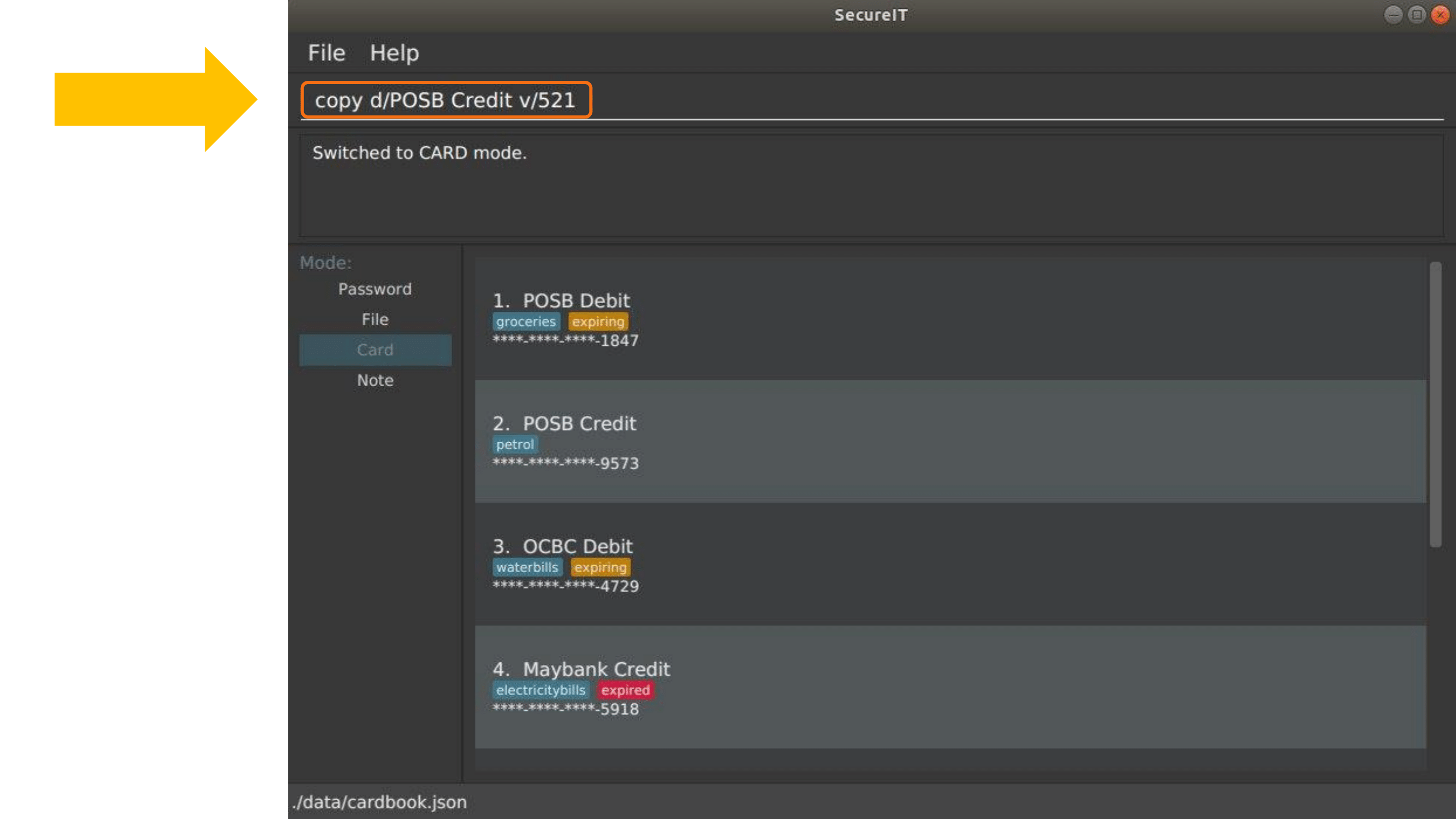
The result box will display the message as shown.
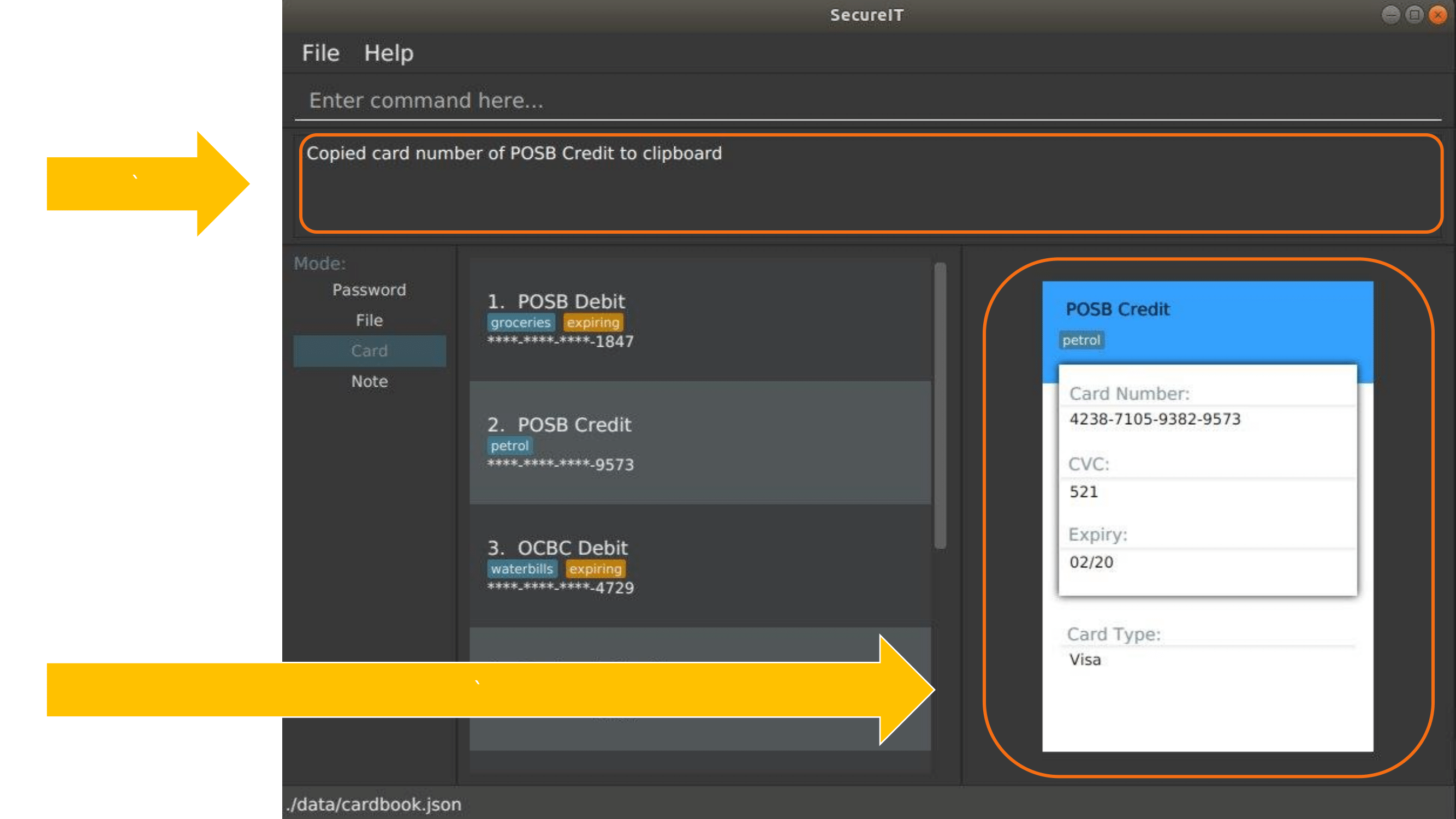
Upon pasting from your clipboard, the card number of your desired card should appear.
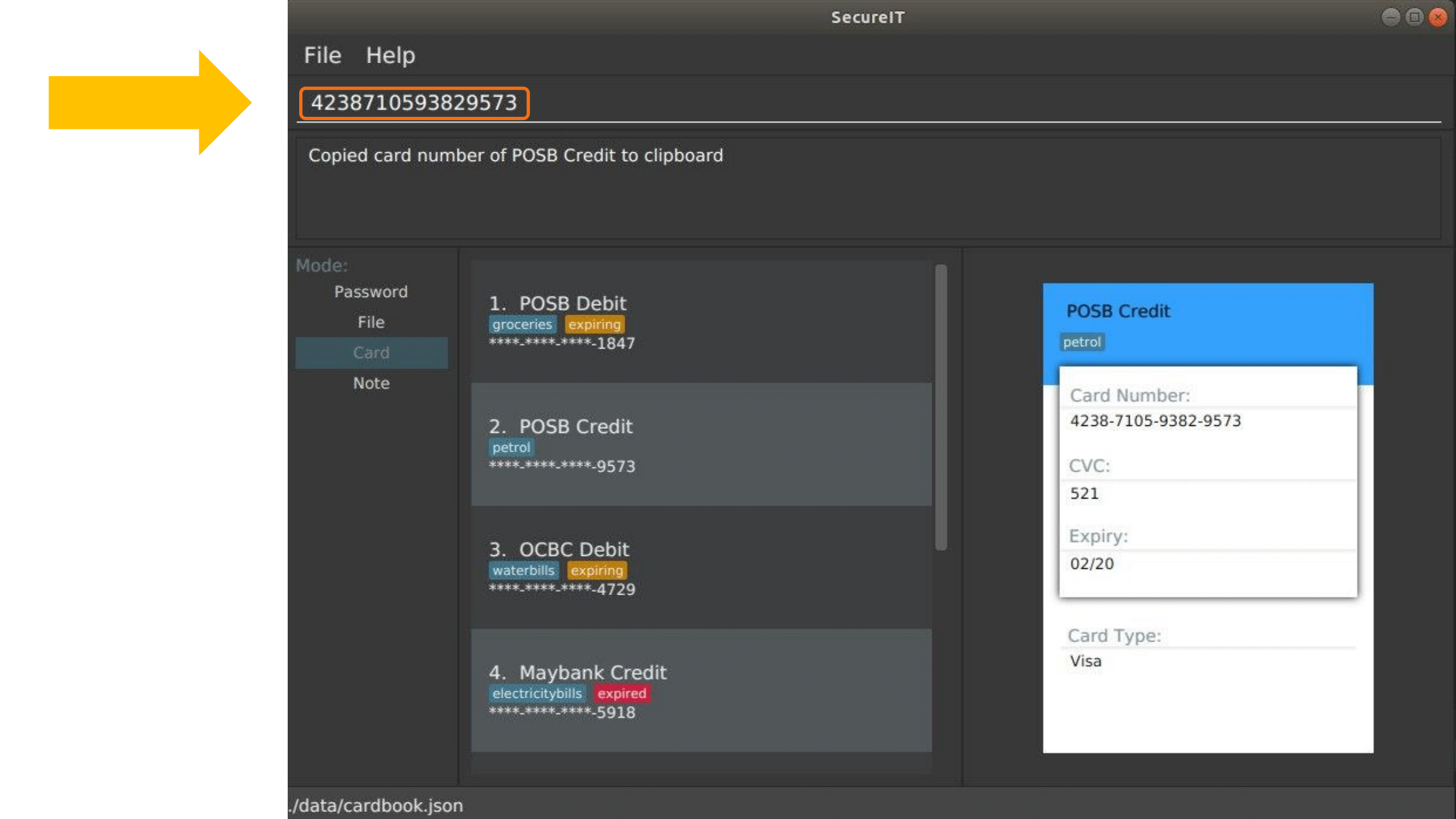
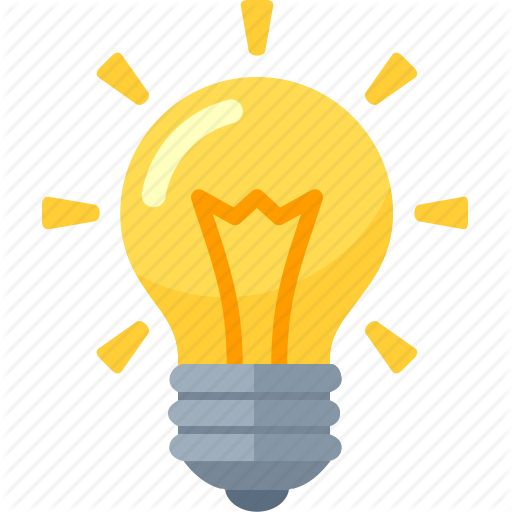
Note:
-
This command only copies the specified card number, and not the CVC. This is because users are expected to remember their CVC so that their card security is not compromised.
Deleting a card: delete
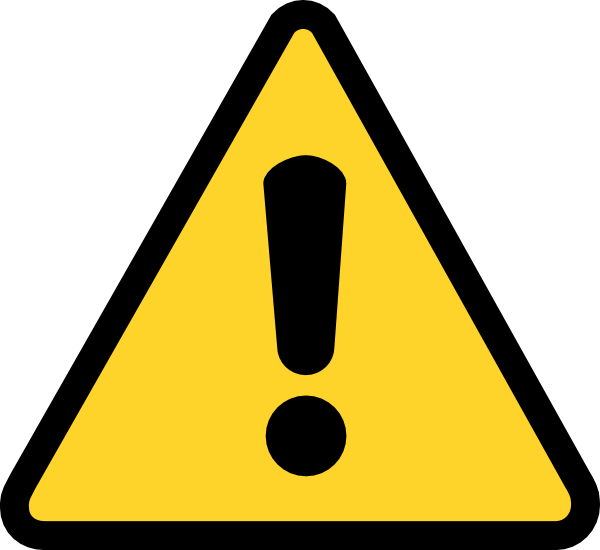
Warning
-
Deleted cards are not recoverable
If your card has expired, you can easily remove it from the application.
Again, you could do this by the card’s description or by its index:
Format: delete DESCRIPTION
OR delete INDEX
Example: delete VisaPOSB
OR delete 1
Type in the command.
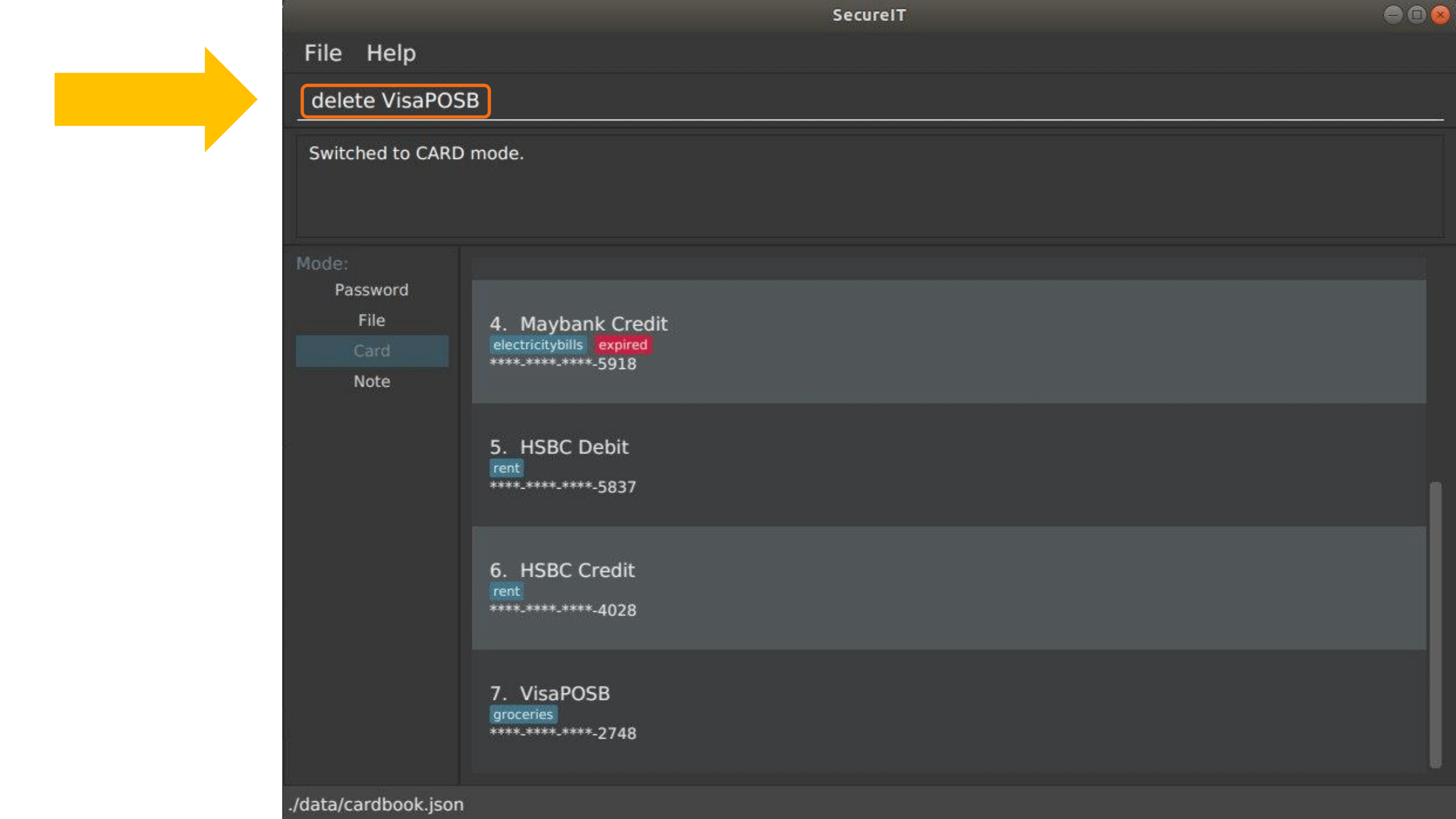
The result box will display the message as shown.
You can see that your card is no longer visible from the list.
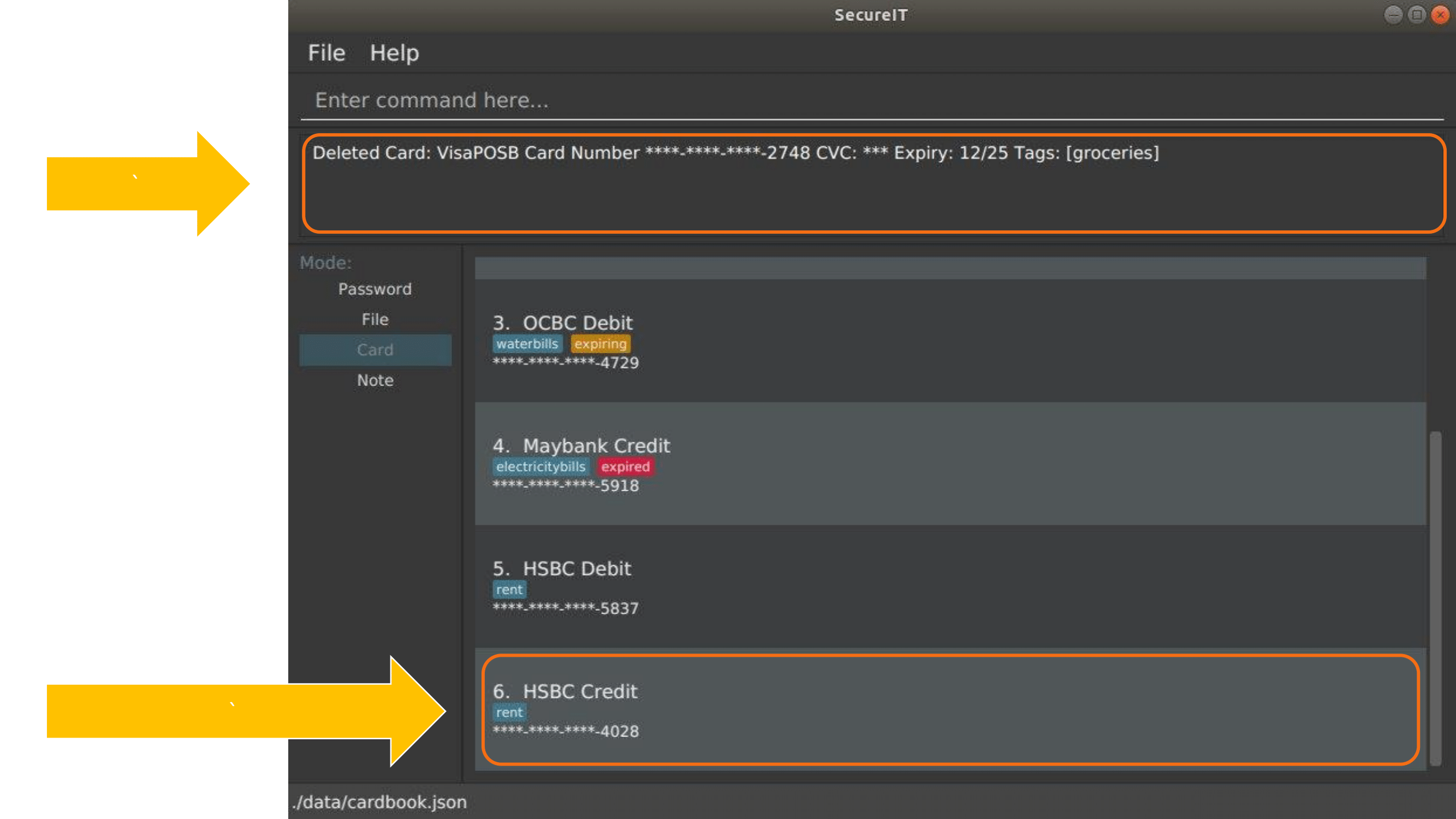
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
Cards Feature
Expiry Validations
When a user tries to add a card, there are various checks to ensure that the expiry entered is valid. As the name suggests, expiry validations are implemented within Expiry
. Additionally, it implements the following operations:
-
ExpiryDate#isValidDate(String test)
— Checks the string against the provided regex to make sure it is a match. -
ExpiryDate#isValidExpiryDate(String test)
— Checks to ensure that it has not past the given expiry date. -
ExpiryUtil#getMonthToExp(String expiry)
— Returns the number of months left until the given expiry.
ExpiryDate#isValidExpiryDate(String test)
is implemented using ExpiryUtil#getMonthToExp(String expiry)
to ensure that the number of months left is positive.
The other operations are used in ParserUtil#parseExpiryDate(String expiryDate)
to ensure that user input passes these validations, before allowing the card to be added to the list. Upon startup of the app, the repopulation of data also checks against these validations to ensure that there are no illegal values stored in the list.
Given below is an example usage scenario and how the validations behave at each step. The following sequence diagram shows how the expiry validations work.
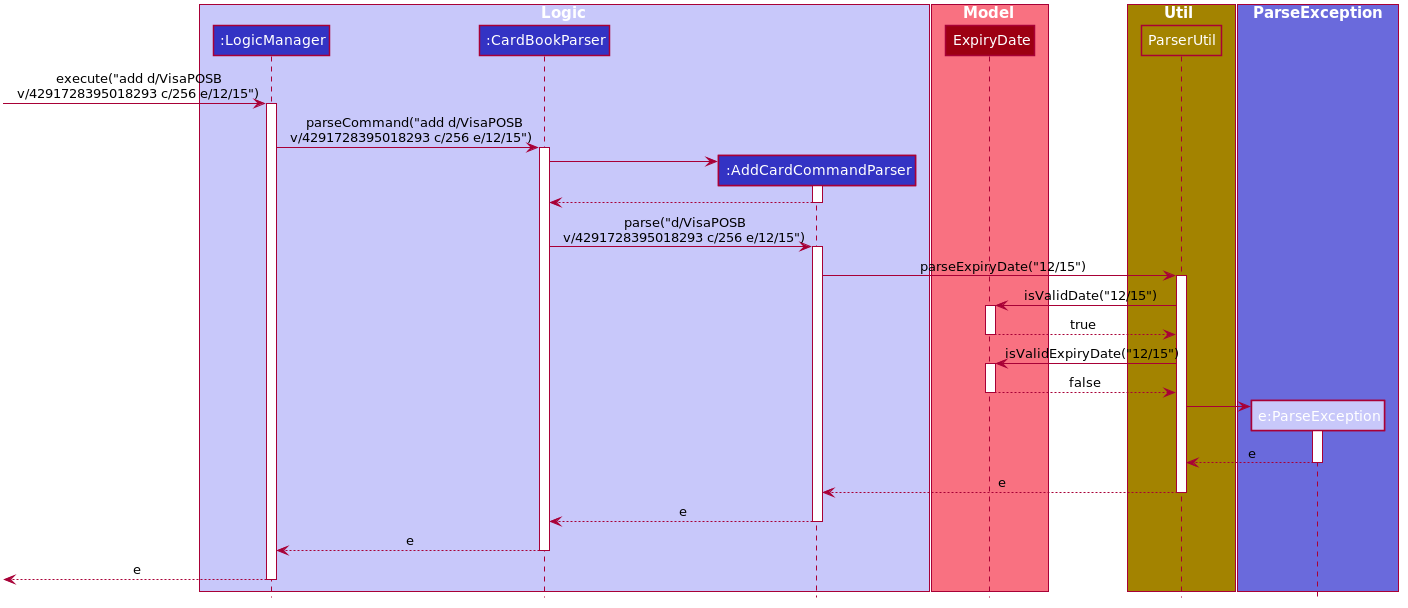
Step 1. The user executes add d/VisaPOSB v/4291728395018293 c/256 e/12/15
command to add a new card named 'VisaPOSB' in the card book. The CardBookParser#parseCommand(String userInput)
calls AddCardCommandParser#parse(String args)
and the command parser tries to breakdown the string in to various parameters.
Step 2. The AddCardCommandParser
calls ParserUtil#parseExpiryDate(String expiryDate)
, which first calls ExpiryDate#isValidDate(String test)
to check its validity, which returns true.
Step 3. The ParserUtil#parseExpiryDate(String expiry)
then calls ExpiryDate#isValidExpiryDate(String test)
, which calls ExpiryUtil#getMonthToExp(String expiry)
. However, -47
is returned and the condition in ExpiryDate#isValidExpiryDate
returns false
.
Step 4. A ParseException
is thrown from ParserUtil#parseExpiryDate(String expiryDate)
and the error message is shown on the result box.
Expiry Notification
The expiry notification is facilitated by ExpiryDisplay
. It exists as an attribute within MainWindow
and obtains the required expiring cards list from LogicManager
. This is internally stored as expiringCards
. Additionally, it implements the following operations:
-
LogicManager#getExpiringCardList()
— Returns the list of expiring cards. -
ExpiringCard#of(Card card)
— Creates an ExpiringCard given card details. -
ExpiryDisplay#hasCards()
— Returns true if there are expiring cards. -
MainWindow#handleExpiry()
— Pops up a notification if there are expiring cards upon startup of the app.
The following sequence diagram shows how the expiry notification shows up.

Step 1. The user opens the app and a new MainWindow
is created. The logic
in MainWindow` calls LogicManager#getExpiringCardList()
to obtain a list of expiring cards.
Step 2. MainWindow
creates a new instance of ExpiryDisplay
using the list of expiring cards.
Step 3. The UiManager
calls MainWindow#handleExpiry()
, and the MainWindow
checks if there are expiring cards with ExpiryDisplay#hasCards()
.
Step 4. The call returns true
and MainWindow
checks if the notification is showing with the call ExpiryDisplay#isShowing()
.
Step 5. This call returns false
and MainWindow
does a final call ExpiryDisplay#show()
to render the expiry notification.
Design Considerations
Aspect: Implementation of expiry tagging system
-
Alternative 1 (current choice): Check for expiring and expired cards and add respective tags upon startup.
-
Pros: Adds the tag in real time on startup, removing the concern of updating tags.
-
Cons: Startup time may take longer due to extra processing of information.
-
-
Alternative 2: Add respective expiring and expired tags upon detection of nearing expiry.
-
Pros: Users can visibly see the addition of expiry tags when cards are expiring.
-
Cons: There will be a need to update expiring tags to expired tags, after the card has expired. This may lead to potential bugs.
-
The reason why we chose alternative 1: It is much easier to code, and it is less susceptible to unforeseen circumstances such as a card having both expiring and expired tags.
Aspect: Deciding when the expiry notification should appear
-
Alternative 1 (current choice): Notification should appear upon startup.
-
Pros: User is immediately warned of their expiring cards and is able to take the necessary actions required.
-
Cons: Potentially disruptive as notification would appear every time the user starts up the app, as long as expiring cards exists.
-
-
Alternative 2: Notification should appear upon navigating to card book.
-
Pros: User can immediately tell which cards are in need of attention.
-
Cons: Expiring cards may be left unnoticed if user does not explicitly navigate to the card book.
-
The reason why we chose alternative 1: The main purpose of notifications is to remind users to take action and users can subsequently delete the card so that the notification stops appearing.